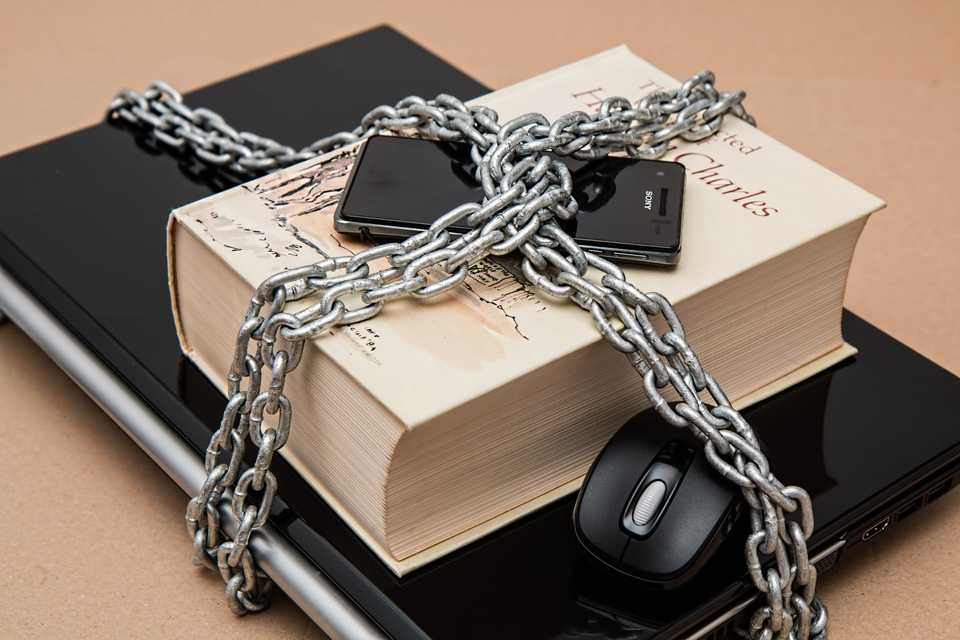
We’ve previously introduced the notion of the user. We’ve also previously removed the security in our Spring-Boot application for the development. However, at some point, the security needs to be introduced again, and we need to know who accesses what. Knowing who is requesting the data is extremely important, especially since we’re handling very sensitive information.
In this post, we’ll cover some of the most common authentication and authorization methods, and then come to the conclusion why I choose OAuth to be the authentication protocol/layer for our application landscape. In a subsequent post, I will then implement OAuth with Google for the Kotlin backend, and after this, in yet another post, I will link the React frontend to this, so that the user will have a seamless experience logging in with his Google account.
The Auths
I have written a lot about Authentication and Authorization in the past. I’ll quickly cover what the differences are here. It seems fairly obvious what Authentication is - yet somehow it’s often mistaken to also contain Authorization, especially when drilling down into the nitty-gritty.
Authentication
Authentication is when an entity, for us a user, proves his identity. It shows us who they really are. This could be done by providing some information only they may have, such as a username and a password, or a token, or anything similar. Authentication can therefore be provided by other trusted sources, such as Google, Facebook, Github, Linkedin, etc. We don’t need to know how they authenticated against them, but we do trust these parties’ word that this user is who they claim to be.
Authorization
Authorization is very different in this regard. Here, it is determined whether the user has the right to receive the information or perform the action they’d like to. It is essentially requiring a successful authentication, as we cannot authorize anything without knowing who we’re dealing with.
So, while authentication could be outsourced to other providers, authorization cannot. Google or Facebook do not know whether we should allow the user to perform a certain action. We still need to determine whether user X can access account Y, or whether that user should be considered an admin and blacklist a user, or anything like that. Authorization will always be the responsibility of the application itself.
Why they’re often used interchangeably
The Auths go hand in hand. Just authenticating someone doesn’t do anybody much good. Authorizing actions without authentication is also impossible. For most operations to be successful, both auths need to be completed beforehand.
There are exceptions, of course. A web shop should ideally NOT require either - the potential customers want to see the wares without having to provide credentials. When the customer wants to actually buy something though, it is key that they, and only they, can perform said action.
Something that always confused me though, is the distinction between the HTTP status codes 401 and 403. If we really think about it though, they are exactly the difference between authentication and authorization.
- 401 Unauthorized is the status code to return when the client provides no credentials or invalid credentials
- 403 Forbidden is the status code to return when a client has valid credentials but not enough privileges to perform an action on a resource
If we now look at these codes, it is clear why they are often used incorrectly - it is the naming. 401 is not really unauthorized, it really is unauthenticated. If this was the actual name of it, I’d never be confused about them ever again! :)
Main authentication methods
The most common authentication methods in Software development are the following:
Basic Authentication
This is perhaps the easiest, but definitely one of the least secure ways for authentication. It passes a value in an Http header called Authorization that contains the word Basic, followed by the username and password encoded in Base64. It should never be used without https, and never in production.
An example would be Authorization: Basic dXNlcjpteVBhc3N3b3Jk
, which would be for username user
and password myPassword
.
API Keys
While API keys are quite widely used, it is still not a great way for authentication. It is mostly used in server-to-server communication, where a third party generates an API key, and you send that key in the header (ideally, it can technically be found in any place, even as unencoded query parameters…).
One of its major advantages is its simplicity. Not even encoding or decoding is required. In cases where security is not the main concern and only READ operations are allowed, this may even be a good solution. For instance, to track stock exchange values, a server/application could identify itself towards another server using an API key.
The most obvious drawback is of course again its simplicity. If this value is not safely stored, anybody can use it for their own purposes also.
OAuth (2.0)
I will not go into the differences between OAuth 1.0 and 2.0, as it’s not really important for our use case in my opinion.
I believe OAuth 2.0 to be the best option for identifying personal accounts. How it works will be described in more detail below.
OpenID Connect
OpenID is technically very similar to OAuth2.0 (it is based on its protocol), but it focuses more on the authentication part, so it will provide information on who you are on your behalf. It can accomplish many of the same things as OAuth can. One of the main differences in features though is that OpenID provides an assertion of identity whereas OAuth is more generic, provides a token, which can then be used to ask the provider questions.
JWT
JSON Web Tokens, in short, JWT, is another open standard, in which tokens are signed using digital keys. This can be symmetric (should not be used in production), or asymmetric, where the private key of the server signs the token and ships it with the public key.
OAuth
What is OAuth
OAuth stands for Open Authorization and is an open-standard authorization protocol or framework that provides applications the ability for secure designated access. This means that a user can give us the right to handle his or her information, maybe access their facebook account and post something, without them giving us their facebook password. This massively reduces the risk on the consumer side - if our application ever gets breached, their facebook account is still as secure as before.
OAuth doesn’t share password data. It instead works using authorization tokens. Essentially, the applications can communicate with one another using the token that was signed by the Authenticator (e.g. Google), and each application then verifies the veracity of this token upon every request with the Authenticator.
The simplest example of this will be our use case. We will ask the user whether they want to sign in on our site with the login of another website. This is us asking the user for authorization to use his token, and them authenticating through that token. It kind of takes care of both the auths.
Advantages of OAuth
OAuth is often also called modern authentication, so that’s already a pointer as to it not being a legacy solution, such as Kerberos for example would be.
However, the main advantage of OAuth is, in my opinion, that it is extremely secure for the user. As outlined above, even if the case of a breach, they are not risking anything additional.
On top of this, there are quite a few OAuth providers out there. The most common examples are Google and Facebook, but as mentioned before, Github and LinkedIn are other options, and there are many more. Once the first integration has been completed, I am counting on the fact that future integrations will be much less time-consuming.
Finally, pretty much the entire world already has at least one of these accounts, so the potential audience is enormous. I know that, at least speaking for myself, using a new application that provides these login options is much more convenient than coming up with yet another login name and password. All it requires is one simple click - the smallest possible hurdle that any user can safely jump.
In conclusion, I am hoping that OAuth will give the necessary security for both the consumer and us, and at the same time, provide an extremely convenient option for the user.
Conclusion
So, this is the reasoning